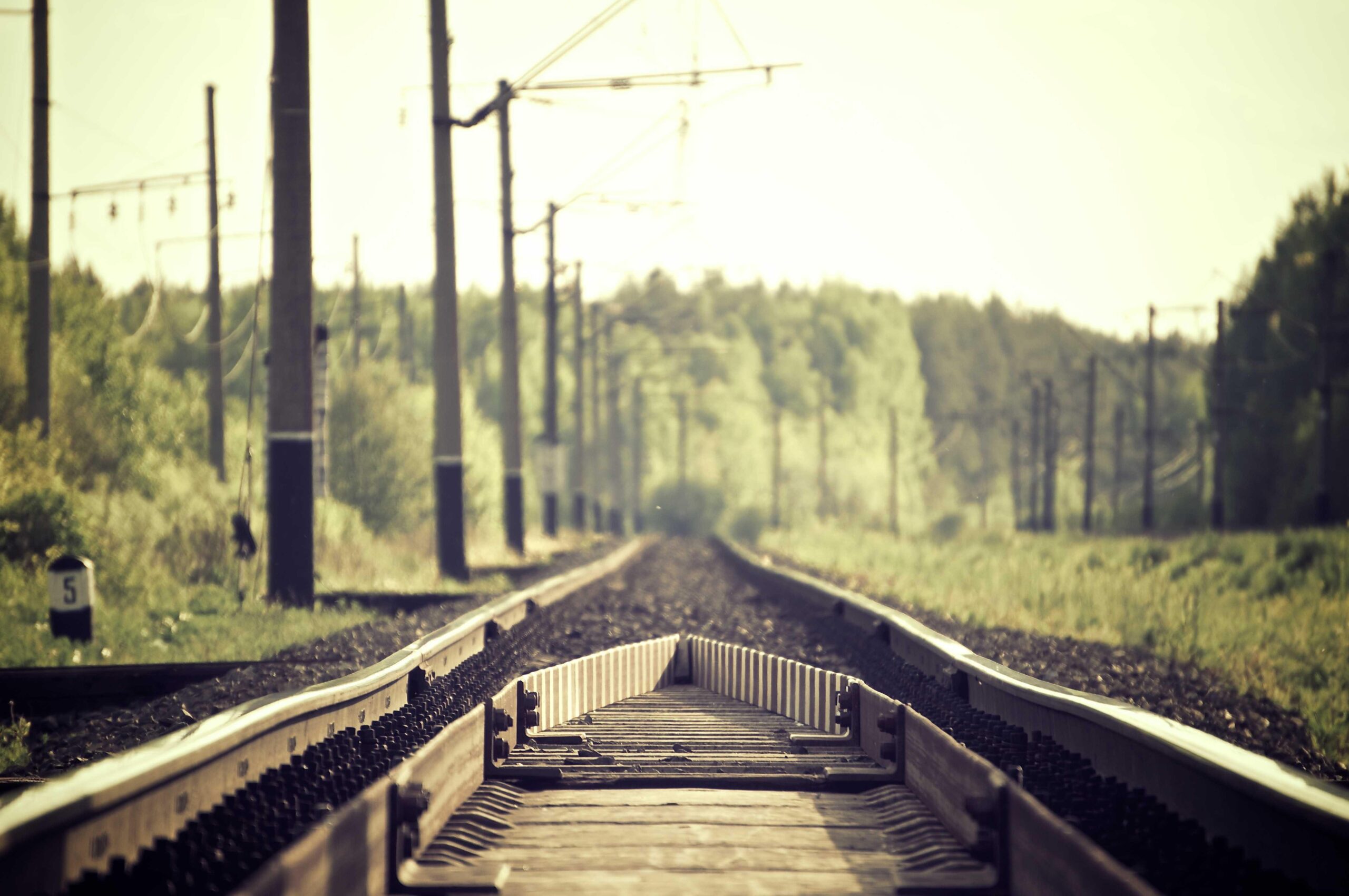
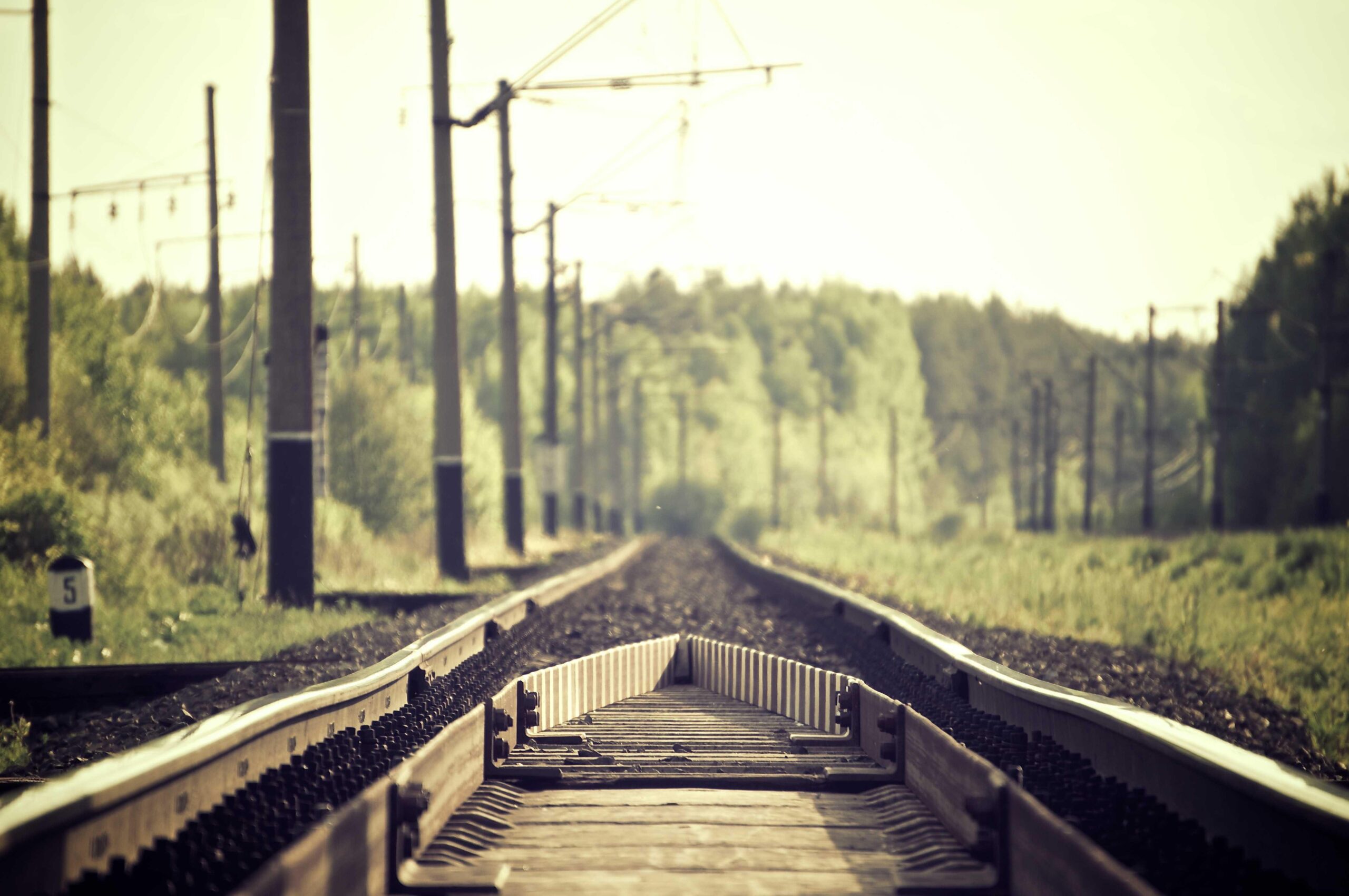
In this step, we’ll delve into some additional advanced topics in Go, including web development, database access, and working with external packages.
Web Development with Go
Go provides a robust ecosystem for building web applications. You can use the standard library’s “net/http” package to create HTTP servers and handle web requests.
Here’s a basic example of a web server:
package main
import (
"net/http"
"fmt"
)
func handler(w http.ResponseWriter, r *http.Request) {
fmt.Fprintln(w, "Hello, Go Web!")
}
func main() {
http.HandleFunc("/", handler)
http.ListenAndServe(":8080", nil)
}
Go also offers popular web frameworks like Gin, Echo, and Beego to simplify building web applications with features like routing, middleware, and more.
Database Access
Go has excellent support for working with databases. You can connect to various databases such as MySQL, PostgreSQL, SQLite, and NoSQL databases like MongoDB. The “database/sql” package is a standard interface for database access.
Here’s a simplified example for connecting to a SQLite database:
package main
import (
"database/sql"
_ "github.com/mattn/go-sqlite3"
"log"
)
func main() {
db, err := sql.Open("sqlite3", "mydb.db")
if err != nil {
log.Fatal(err)
}
defer db.Close()
// You can execute SQL queries and interact with the database here.
}
emember to import the appropriate database driver package for the database you’re using.
Working with External Packages
Go has a vast ecosystem of third-party packages available on the Go Package Repository (pkg.go.dev). You can easily integrate these packages into your projects using the import
statement.
For example, if you want to use a JSON library, you can import the "encoding/json"
package:
import "encoding/json"
You can install external packages with the go get
command. For example:
go get github.com/some/package
Ensure you document and manage your project’s dependencies with a go.mod
file.
Cross-Compilation
Go allows for cross-compilation, meaning you can build executables for different platforms and architectures from a single codebase. You can do this using the GOOS
and GOARCH
environment variables.
For example, to build a Windows executable on a Linux machine, use:
GOOS=windows GOARCH=amd64 go build -o myapp.exe
This is especially useful when distributing your Go applications on multiple platforms.
Interfaces in Go
In Go, an interface is a type that defines a set of method signatures. It’s a way to specify behavior, rather than structure. Any type that implements all the methods of an interface is said to satisfy that interface. For example:
type Shape interface {
Area() float64
}
type Circle struct {
Radius float64
}
func (c Circle) Area() float64 {
return math.Pi * c.Radius * c.Radius
}
func main() {
var shape Shape
shape = Circle{Radius: 5}
area := shape.Area()
fmt.Println("Area:", area)
}
Here, Circle
satisfies the Shape
interface by implementing the Area
method.
Goroutines
Goroutines are lightweight threads in Go, and they are a fundamental part of concurrent programming. You can start a new goroutine using the go
keyword. For example:
func main() {
go sayHello()
time.Sleep(time.Second)
}
func sayHello() {
fmt.Println("Hello, Goroutine!")
}
In this example, sayHello()
is executed concurrently with the main program.
Channels
Channels are used for communication and synchronization between goroutines. They allow goroutines to send and receive data. You can create a channel like this:
ch := make(chan int)
Here’s an example of using channels for communication:
func main() {
ch := make(chan string)
go func() {
ch <- "Hello, Channel!"
}()
msg := <-ch
fmt.Println(msg)
}
In this example, a message is sent from one goroutine to another via a channel.
Now, with these concepts in mind, we can explore advanced concurrency in Go in next Blog.
Can you be more specific about the content of your article? After reading it, I still have some doubts. Hope you can help me.