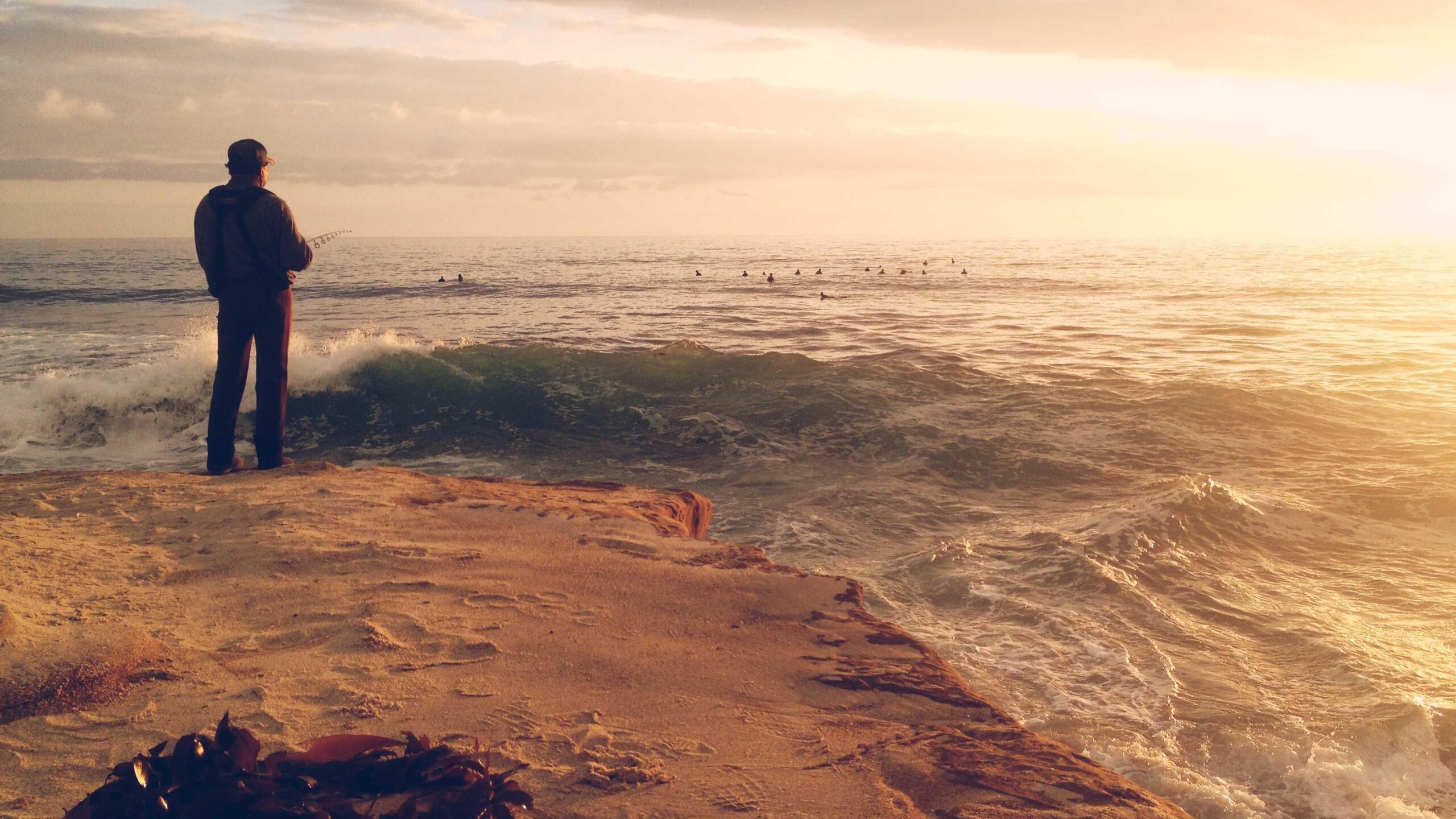
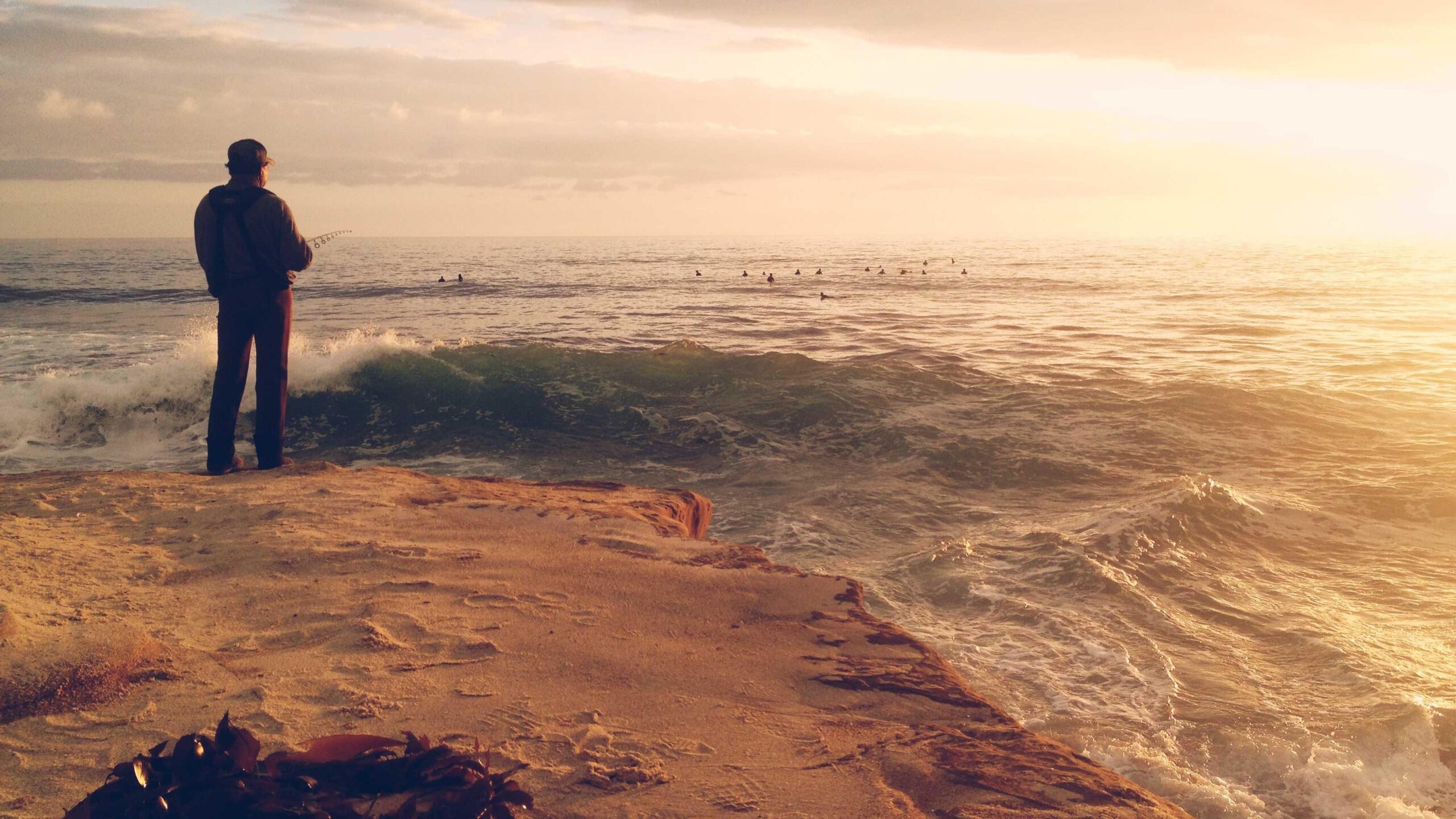
To start with, Go is an open-source programming language originally developed by a team at Google, which includes Ken Thomson, creator of UNIX and C, and enhanced by many contributors from the open-source community.
As Moore’s Law is reaching the end of its lifecycle, the need for concurrency is rising, and consequently, the need for a programming language that enables effective implementation of concurrent programs is rising too. For this reason, Go has become one of the most popular languages in recent times.
Go programming language was designed by Google to solve Google’s problem with respect to developing software at scale. Google develops works on millions of lines of code on a daily basis, mostly written in C++ and lots of Java and Python.
The language was designed by and for people who write — and read and debug and maintain — large software systems.
It has modern features like garbage collection from Java, and it also takes advantage of powerful multi-core computer capabilities with built-in concurrency support, again similar to Java.
Because of these excellent features and speed and reliability, Golang is getting a lot of traction from different types of developers around the world. Many of them use it for application development, infrastructure automation, and cloud-native coding.
So Lets start with the Basics in this Chapter, we will deep dive into Primitives
Primitives
In Go, primitives are the basic data types used to represent simple values like numbers, text, and boolean values. Here’s an overview of the primitive data types in Go:
Numeric Types:
- int: Represents signed integers. The size (bits) of int depends on the platform. For most systems, it’s either 32 or 64 bits.
- uint: Represents unsigned integers. Like int, its size depends on the platform.
- int8, int16, int32, int64: Signed integers with specific sizes (8, 16, 32, or 64 bits).
- uint8, uint16, uint32, uint64: Unsigned integers with specific sizes.
- float32: Represents 32-bit floating-point numbers.
- float64: Represents 64-bit floating-point numbers.
- complex64, complex128: Complex numbers with 64-bit or 128-bit parts.
String Type:
- string: Represents a sequence of characters. Strings are immutable in Go, meaning you can’t change their contents once created.
Boolean Type:
- bool: Represents boolean values, which can be either
true
orfalse
.
Character Type:
- byte (alias for uint8): Represents a single byte, often used to represent ASCII characters.
- rune (alias for int32): Represents a Unicode code point and is used for representing characters and symbols from all languages.
package main
import "fmt"
func main() {
// Numeric types
var x int = 42
var y float64 = 3.14
var z complex128 = 1 + 2i
// String type
name := "John"
// Boolean type
isTrue := true
// Byte and Rune types
char := 'A' // Rune representing the character 'A'
asciiChar := byte('B') // Byte representing the ASCII character 'B'
fmt.Println("Numeric Types:")
fmt.Println("int:", x)
fmt.Println("float64:", y)
fmt.Println("complex128:", z)
fmt.Println("\nString Type:")
fmt.Println("string:", name)
fmt.Println("\nBoolean Type:")
fmt.Println("bool:", isTrue)
fmt.Println("\nCharacter Types:")
fmt.Println("rune:", char)
fmt.Println("byte:", asciiChar)
}
In the above example:
- We declare variables of various primitive types and assign values to them.
- Go supports type inference, so you can use
:=
to declare and initialize variables without explicitly specifying their types. - We print the values of these variables using
fmt.Println()
.
This code demonstrates the basic usage of primitive data types in Go. In Future chapters we will Learn more about arrays, maps, loops and much more.
Can you be more specific about the content of your article? After reading it, I still have some doubts. Hope you can help me.
Thank you for your sharing. I am worried that I lack creative ideas. It is your article that makes me full of hope. Thank you. But, I have a question, can you help me?
Thank you for your sharing. I am worried that I lack creative ideas. It is your article that makes me full of hope. Thank you. But, I have a question, can you help me?
Can you be more specific about the content of your article? After reading it, I still have some doubts. Hope you can help me.