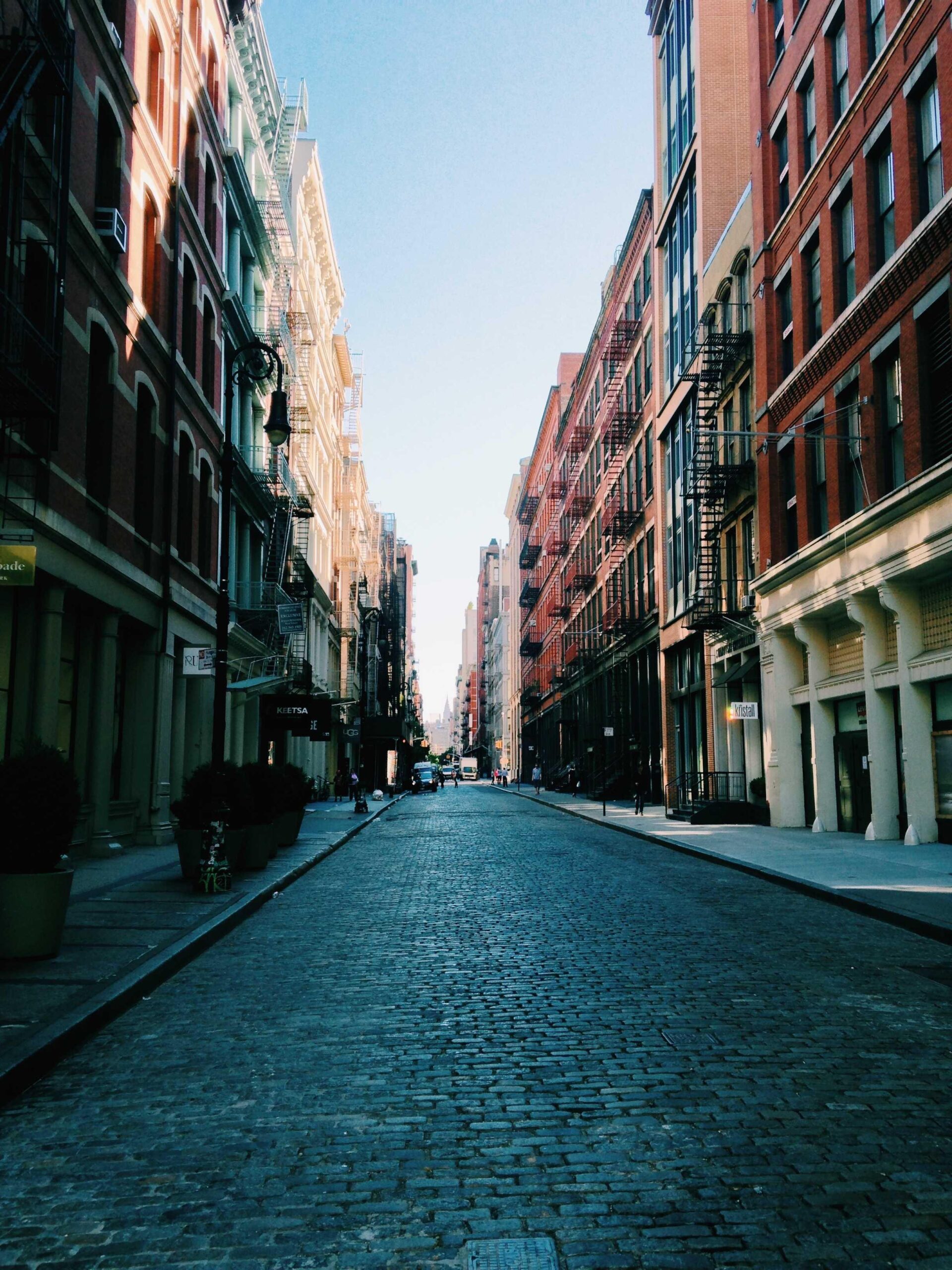
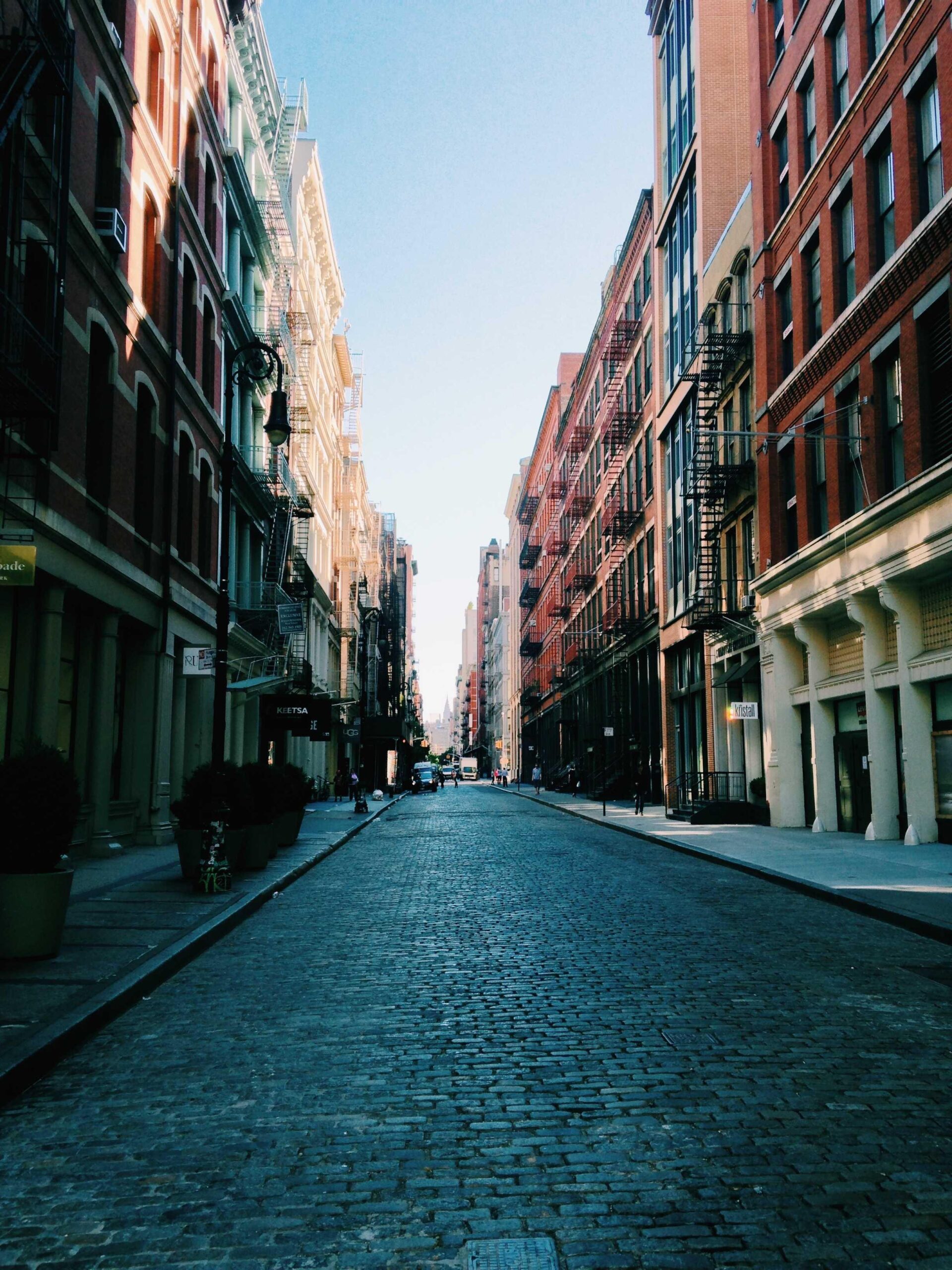
Variables in Go
In Go, you declare variables using the var
keyword followed by the variable name and its data type. Here’s how you declare and initialize variables:
var age int = 25
var name string = "Alice"
You can also use type inference to let Go determine the variable’s type:
age := 25
name := "Alice"
Data Structures
Go offers several built-in data structures:
Arrays:
- Fixed-size collections of elements of the same type.
var numbers [5]int
numbers[0] = 1
numbers[1] = 2
// ...
Slices:
- Dynamic, resizable arrays.
mySlice := []int{1, 2, 3, 4, 5}
Maps:
- Key-value pairs.
myMap := make(map[string]int)
myMap["apple"] = 10
myMap["banana"] = 5
Structs:
- Custom data structures that group variables of different types.
type Person struct {
Name string
Age int
}
person := Person{Name: "Bob", Age: 30}
Control Flow
Go provides common control flow constructs:
- Conditional Statements:
if
,else if
, andelse
for conditional execution
if age >= 18 {
fmt.Println("You're an adult.")
} else {
fmt.Println("You're a minor.")
}
2. Loops:
for
for loops.
for i := 0; i < 5; i++ {
fmt.Println(i)
}
3. Switch Statements:
- Used to perform different actions based on a variable’s value.
switch day {
case "Monday":
fmt.Println("It's Monday.")
case "Friday":
fmt.Println("It's Friday!")
default:
fmt.Println("It's another day.")
}
Functions
Functions are a fundamental part of Go. You can define and call functions like this:
func add(x, y int) int {
return x + y
}
result := add(3, 5)
Packages
Go is built around the concept of packages. You can import and use functions and types from other packages to build your applications. For example, the fmt
package is used for input and output.
import "fmt"
func main() {
fmt.Println("Hello, Go!")
}
Error Handling
Error handling is crucial in any programming language, and Go provides a simple and effective way to handle errors using the built-in error
interface and the errors
package.
import "errors"
func divide(x, y float64) (float64, error) {
if y == 0 {
return 0, errors.New("division by zero")
}
return x / y, nil
}
result, err := divide(10.0, 0.0)
if err != nil {
fmt.Println("Error:", err)
} else {
fmt.Println("Result:", result)
}
Interfaces
Interfaces in Go enable you to define a set of methods that a type must implement. This allows for polymorphism and helps create more flexible and reusable code. For example:
type Shape interface {
Area() float64
}
type Circle struct {
Radius float64
}
func (c Circle) Area() float64 {
return math.Pi * c.Radius * c.Radius
}
func PrintArea(s Shape) {
fmt.Printf("Area: %f\n", s.Area())
}
circle := Circle{Radius: 5}
PrintArea(circle)
Concurrency
Go is known for its strong support for concurrent programming. You can create concurrent programs using Goroutines and Channels. Goroutines are lightweight threads, and Channels allow communication between them.
func main() {
messages := make(chan string)
go func() {
messages <- "Hello, Go!"
}()
msg := <-messages
fmt.Println(msg)
}
Concurrency can help you build efficient programs that can perform tasks concurrently without blocking the main execution.
Packages and Dependency Management
Go uses a module system for dependency management. You can create your own modules and import third-party packages.
To create a new module, use the go mod
command:
go mod init yourmodule
To install and use a third-party package, use go get
:
go get github.com/example/package
Error Handling and Panic
In Go, panic
is used to terminate a program due to unrecoverable errors. It should be used sparingly. You can recover from panics using the recover
function, but it’s typically used for cleanup, not error handling.
func example() {
defer func() {
if r := recover(); r != nil {
fmt.Println("Recovered from a panic:", r)
}
}()
// Some code that might panic
}
### Unit Testing
Go has built-in support for unit testing. You can write test functions in files ending with `_test.go` and use the `testing` package.
```go
func add(x, y int) int {
return x + y
}
func TestAdd(t *testing.T) {
result := add(3, 5)
if result != 8 {
t.Errorf("Expected 8, but got %d", result)
}
}
You run tests with the go test
command.
Your article helped me a lot, is there any more related content? Thanks!
Thank you for your sharing. I am worried that I lack creative ideas. It is your article that makes me full of hope. Thank you. But, I have a question, can you help me?